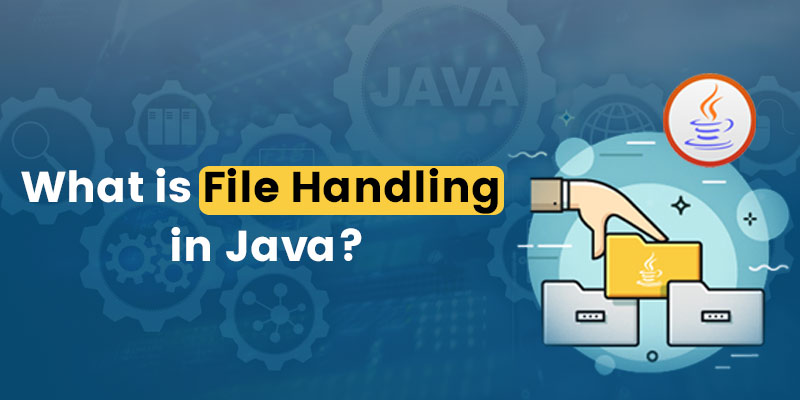
Java has revolutionized software development, empowering countless developers to build scalable, robust, and platform-independent applications. An essential component of Java programming is file handling, which involves interacting with external files, such as reading or writing files on a disk. In this blog, we will explore the fundamental concepts of file handling in java, discussing key concepts and demonstrating common file manipulation tasks. FITA Academy provides comprehensive and professional JAVA Training in Chennai, with extensive industry knowledge spanning several decades, and is dedicated to assisting you in mastering the fundamental concepts of Java file handling.
File Handling
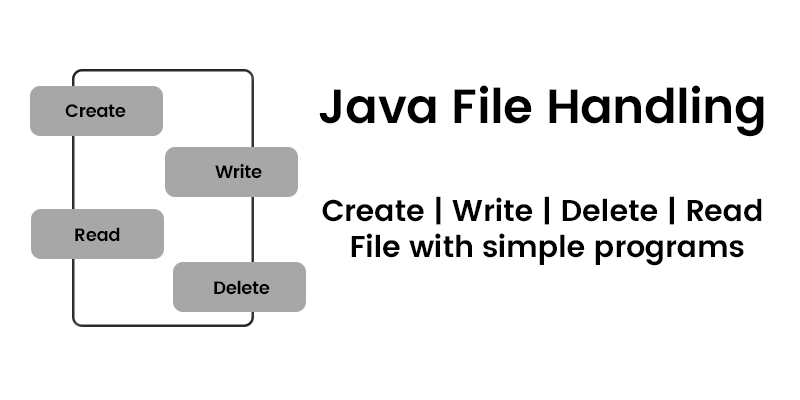
File handling enables users to interact with files, manipulate file attributes, perform operations such as reading or writing data, and manage file streams. The java.io file class specifically caters to handling files in different formats. File handling encompasses essential tasks such as creating, reading, writing, deleting, and manipulating file attributes for directories and files. Java offers various methods and classes within java.io and java.nio.file packages to facilitate file-related operations. To utilize the file class in java, users simply need to create an object of the respective class and specify the desired filename or directory name.
Reading from a Files
Both reading and writing files in Java involve utilizing file channels for efficient data transfer between files and buffers. The user uses a channel object obtained from a FileInputStream object to read a file. Subsequently, the user can use a FileChannel object acquired from a RandomAccessFile object to perform reading and writing operations on the same file. It is important to note that a FileInputStream object encapsulates a file intended for reading.
Example
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class FileReaderExample {
public static void main(String[] args) {
try {
File file = new File("filename.txt");
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
// Process each line of the file
System.out.println(line);
}
scanner.close();
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
}
}
}
Writing to a file
To know about how to write a file in java, let’s say that the user loads the file, which has been created, into one or more buffers. A method is invoked on the corresponding object to write the data to the file which is encapsulated within the file stream. The user utilizes the write() method for a file channel to initiate the writing process. This method writes the data stored in a single ByteBuffer object to the file. The number of bytes written to the file is determined by the position and limit of the buffer when the write() method is executed. If you are interested to learn more about Java, consider enrolling in Java Training in Bangalore, where students can acquire comprehensive knowledge and understanding of Java's fundamental concepts.
Example
import java.io.FileWriter;
import java.io.IOException;
public class FileWriterExample {
public static void main(String[] args) {
try {
FileWriter writer = new FileWriter("filename.txt");
writer.write("Hello, world!");
writer.close();
System.out.println("Data written to the file successfully.");
} catch (IOException e) {
System.out.println("An error occurred: " + e.getMessage());
}
}
}
Creating a file
To create a new file using File handling, you can leverage the File class in combination with the FileOutputStream class. Start by specifying the desired file name and assigning a string value to the fileName variable. Create a file object by invoking the File constructor with the file name as the parameter. The next step is to employ the createNewFile() method of the File object to generate a new file. This method returns true if the file is created successfully. However, if the file already exists, the method will return false.
Example
import java.io.File;
import java.io.IOException;
public class CreateFileExample {
public static void main(String[] args) {
try {
File file = new File("filename.txt");
boolean created = file.createNewFile();
if (created) {
System.out.println("File created successfully.");
} else {
System.out.println("File already exists.");
}
} catch (IOException e) {
System.out.println("An error occurred: " + e.getMessage());
}
}
}
Deleting a file
To delete a file using file handling, you can utilize the file class in java along with the delete() method. To initiate the process, assign a string value to the variable "fileName" that accurately represents the name of the file to be targeted for deletion. Next, create a File object by passing the filename to the constructor. Check if the file exists using the exists() method of the File object. If the file exists, use the delete() method to remove the file. The delete() method provides a boolean return value, indicating true if the file deletion is successful, and false if it is not. Display an appropriate message indicating the outcome of the deletion process. Completing a Java Course in Coimbatore helps students to get a better understanding of how to delete a file Java.
Example
import java.io.File;
public class DeleteFileExample {
public static void main(String[] args) {
File file = new File("filename.txt");
boolean deleted = file.delete();
if (deleted) {
System.out.println("File deleted successfully.");
} else {
System.out.println("File deletion failed or file does not exist.");
}
}
}
Streams
Streams play an important role in file handling in java, facilitating input and output operations. They act as a channel through which data can be read or written, serving as a source or destination for the data. Conceptually, streams represent a flow of bytes, representing the continuous series of data entering or exiting a program. Streams are the fundamental representation of input or output, serving as the medium for data transfer. We can efficiently write data into the stream or read specific data with streams. Visualizing the data stream as a sequence of bytes flowing into or out of the program helps understand the data flow and processing.
Example for Reading from a file using streams
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
public class FileStreamReaderExample {
public static void main(String[] args) {
try (InputStream inputStream = new FileInputStream("filename.txt")) {
int data;
while ((data = inputStream.read()) != -1) {
// Process each byte of data
System.out.print((char) data);
}
} catch (IOException e) {
System.out.println("An error occurred: " + e.getMessage());
}
}
}
Example for Writing to a file using streams
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class FileStreamWriterExample {
public static void main(String[] args) {
try (OutputStream outputStream = new FileOutputStream("filename.txt")) {
String data = "Hello, world!";
byte[] bytes = data.getBytes();
outputStream.write(bytes);
System.out.println("Data written to the file successfully.");
} catch (IOException e) {
System.out.println("An error occurred: " + e.getMessage());
}
}
}
Input and Output Streams
Input and output streams are essential elements in file handling in java, which provides efficient capabilities for reading and writing files. An output stream can be directed to various devices, such as hard disks or any stream capable of holding a sequence of bytes. It can also be displayed on output screens with unique capabilities. Only characters can be displayed when outputting to the display screen, while graphical content requires specialized support.
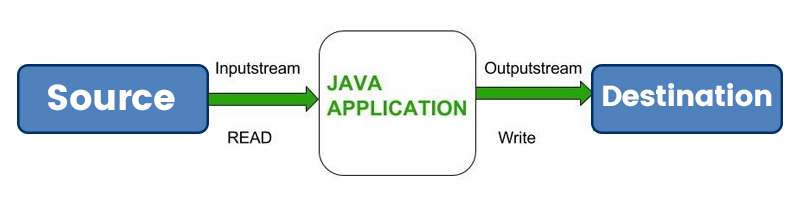
In Java, output streams are vital in writing data to streams, enabling communication with different devices or destinations. Whether transmitting data over a network, displaying information on a screen, or writing to a hard disk, output streams provide a versatile mechanism for data transfer. Both Input and OutputStreams are Byte Streams. Enroll in Java Online Course, which teaches Java Concepts in the best professional way and expands your expertise in this field.
Example for Input Stream
import java.io.InputStream;
public class InputStreamExample {
public static void main(String[] args) {
try {
InputStream inputStream = // Obtain the input stream, e.g., from a file or network
int data;
while ((data = inputStream.read()) != -1) {
// Process the data read from the input stream
// ...
}
inputStream.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Example for Output Stream
import java.io.OutputStream;
public class OutputStreamExample {
public static void main(String[] args) {
try {
OutputStream outputStream = // Obtain the output stream, e.g., to a file or network
// Write data to the output stream
// ...
outputStream.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
The Classes for Input and Output
The java.io package provides an extensive set of classes to support streams. For more information about what is stream class in java, let’s say its a fundamental component which represents a sequence of elements that can be processed in a sequential or parallel manner. Acknowledging the logical structure of these classes is very important to use them effectively in the programs. We will explore the class hierarchy, allowing you to grasp how these classes relate and how they can be combined to address various scenarios. java.io contains all the classes for the support of the streams. Both Input and Output streams are Abstract classes.
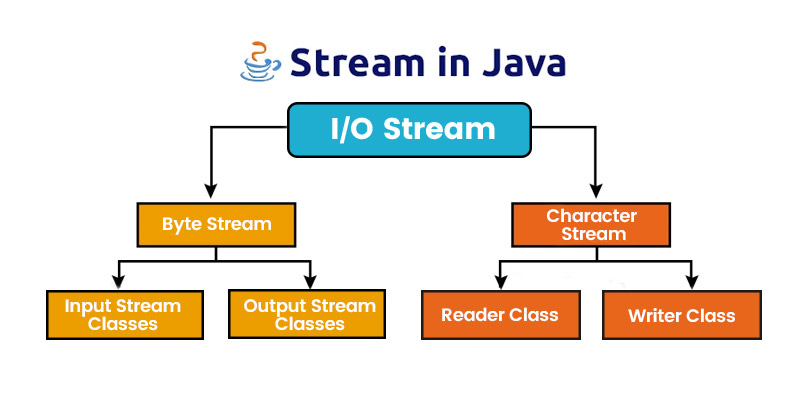
A reader is utilized in Java when there is a need to read character-based data from a data source, such as a file or a stream. A writer is used when there is a requirement to write character-based data to a destination, such as a file or a stream. Both Reader and Writer classes are Character Streams.
Example of Input Stream
// Import the required classes
import java.io.InputStream;
// Create an InputStream object
InputStream inputStream = null;
try {
// Initialize the input stream with a specific source (e.g., a file or network stream)
inputStream = new FileInputStream("file.txt");
// Read data from the input stream
int data;
while ((data = inputStream.read()) != -1) {
// Process the data
System.out.print((char) data);
}
} catch (IOException e) {
// Handle any exceptions
e.printStackTrace();
} finally {
// Close the input stream in the finally block to ensure it gets closed even in case of exceptions
if (inputStream != null) {
try {
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Example of Output Stream
e.printStackTrace();
finally {
// Close the output stream in the finally block to ensure it gets closed even in case of exceptions
if (outputStream != null) {
try {
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
File handling is a fundamental aspect of many applications, enabling us to read, write, and manipulate data in files. So now, we have explored what is file handling in java, covering key concepts, techniques, examples, input and output streams, and stream classes. FITA Academy offers the best Java Course in Madurai for students to help them gain more knowledge about fundamental concepts of Java.