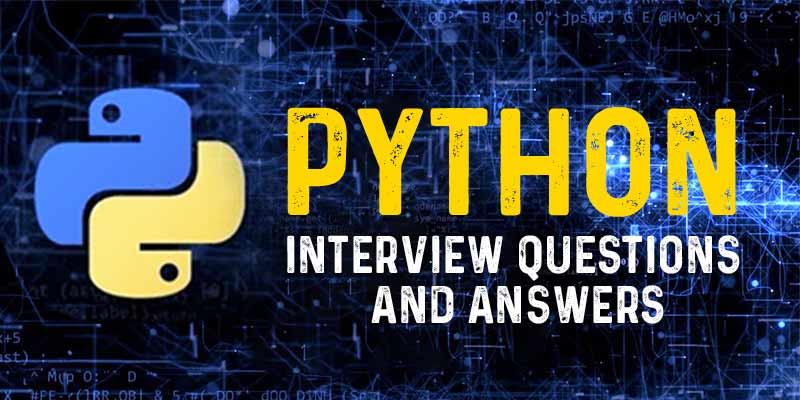
Major organizations prefer using Python for the development of applications because it results in a reduction of production time, speedy development and reduced time-to-market, increased business speed, and boosted competitiveness.
Python is constantly ranked as one of the top choices for developers and also recruiters. To help you impress the recruiters and get hired for the job you want, we present to you 101 Python interview questions and answers.
Python language is being adopted as a career choice by budding developers worldwide due to its versatility and easy coding syntax. Its other features like robust, extensive Library Support, Versatility, etc. have made it immensely popular. The extensive use of Python across many upcoming technologies has increased the likelihood of lucrative job opportunities therefore, the demand for Python professionals has grown.
Since the competition is so strong, it can be a little difficult to clear the interview. We highly recommend you to go through these Python interview questions and answers which are compiled for both beginners and experts. You can even download the Python interview questions and answers pdf.
-
What kind of a language is python, programming, or scripting?
Generally, all scripting languages are considered programming languages. The only difference is that scripting languages are directly interpreted without any compilation. Python is considered among the best programming and scripting languages.
-
List any 5 key features of the Python language.
It is an object-oriented programming language which means it supports classes, objects, and inheritance.
It is a portable language i.e., it allows the one code to run on multiple platforms.
Python is dynamically typed so we don’t need to define any variable types.
It is an interpreted language which means it doesn’t need to be compiled as the code is executed line by line.
Python is a high-level language so we don’t need to remember the system architecture or worry about memory allocation.
-
What is PEP 8?
Python Enhancement Proposal (PEP) is a set of rules documented by Guido van Rossum, Barry Warsaw, and Nick Coghlan in 2001. It specifies the format for python code for maximum readability and provides information about the Python community.
-
What are modules in python?
Modules are program files containing executable Python code, like functions and classes which you want to include in your source code. It helps in logically organizing your code. To create a module save the code you want in a file with the .py extension.
-
What is a namespace in Python?
Python namespace is a system in which every object, variable, or function contains a unique name. It can be considered as a mapping of every name defined to a corresponding object. It can be of 3 types: local, built-in and global.
-
What are Python packages?
A package is a file having a directory-like structure containing various modules and sub-packages. Each python package must contain a file named _init_.py. This file indicates that the directory it contains is a package.
-
What is the use of libraries in python? List any 5
A library can be defined as a collection of modules. The line of difference between python libraries and packages is very thin.
A python library is a reusable block of code which is extremely useful in eliminating the need to write code from scratch. They play a crucial role in the development of machine learning, data science, image, and data visualization. Some of the most important Python libraries are Numpy, Pandas, Math, Matplotlib, Scipy.
-
What is _init_ in Python?
In python, _init_ is a method or a constructor present in every class. Whenever a new object or instance is created, the _init_ method is automatically called for memory allocation.
-
Explain the importance of indentation in Python?
The whitespaces at the beginning of a code line are called indentation. In Python, the indentation of code is extremely important, unlike other languages where indentation is only used for better code reliability.
In Python, indentation is used to specify a block of code. All the lines belonging to the same block of code should contain the same number of line spaces. If you skip the indentation, Python generates an error.
-
What is the scope? What are the different types of scope in Python?
The scope of an object is a block of code where that object remains valid. There are 4 types of scope in python:
Local Scope: Objects remain valid in a particular method.
Global Scope: Object is relevant throughout the code.
Module-level: Global object of the module being used in a program.
Outermost Scope: These are callable built-in names in a program.
-
State 1 similarity and 1 difference between list and tuple.
Both list and tuple are a kind of data type in python which are used to store a sequence of objects/values.
The main difference between them is that lists are mutable i.e., they can be modified even after being created while tuples are immutable so they cannot be altered.
-
What is the self?
It is used to define an instance of a class. It is the first attribute in the instance of any class and is used to access the methods and variables of that class. ‘Self ’ is a name given by default, you can change it to anything you like.
-
State 2 ways using which you can reverse a list.
The first way is by using the reverse function.
Eg. a =[1,2,3]
a.reverse()
The second way is by using a[::-1]. The only difference is that you need to store this in another variable as the reversed changes are not reflected in the original variable.
-
What is the difference between capitalize() and upper() function?
The capitalize() function converts only the first letter of the string to uppercase while upper() converts the whole string to uppercase.
-
How can you separate a string without whitespaces into a list?
a = ‘12345’
l = list(map(int,a))
Output : [1,2,3,4,5]
-
What is a docstring?
A docstring is not a comment but a multiline string containing the documentation of the code. It describes the working of a function or method. These are enclosed between triple quotes.
-
Explain the process of slicing in Python.
Slicing is a way to select a range of items from sequence datatype objects like lists, tuples, and strings. It takes part in an object.
Syntax : [start : stop : steps]
Start defines the beginning of a string from where we want to start the slicing. Its default value is 0.
Stop is the endpoint of the string till where you want to stop. Its default value is the length of the string.
Steps specify the number of jumps to want to take between each slice. Its default value is 1.
Eg: a = [1,2,3,4,5,6,7,8,9,10]
a[1:8:2]
Output : [2, 4, 6, 8]
-
What will be the output of a[4] in list a = [1,2,3,4,5,6,7,8]?
Since the indexing starts from 0, for a[4] we will count from 0 to 4 and fetch the value. So the output will be 5.
-
What is an array? How is it different from the Python list?
An array is a data structure used to store multiple objects at contiguous memory locations. Each element of an array is identified by an array index or key.
But in python, an array can only contain elements of the same data type, unlike lists which can store heterogeneous elements. Since arrays store homogeneous elements they consume considerably less memory as compared to lists. This kind of question is among the important Python Interview Questions and Answers for Freshers.
-
Give an example of the use of the negative index in python.
Negative indices imply that the counting starts from the end of the list. -1 indicates the last element of the list and -4 indicates the fourth last element.
a = [1,2,4,4,5,6]
a[-1 ]
Output = 6
-
What is the difference between remove, del, and pop?
All three of them serve the same purpose with different approaches. Del is a keyword in python while remove and pop are built-in functions. Remove takes the value you want to remove as the argument while del and pop take the index of the item as the argument.
a = [1,2,3,4,5,6,7,8,9,10]
del a[2] // a = [1,2,4,5,6,7,8,9,10]
a.remove(6) // a = [1,2,4,5,7,8,9,10]
a.pop(4) // a = [1,2,4,5,8,9,10]
-
How can you add values to an array in Python?
There are 3 methods by give values can be added to an array, namely append(), extend() and insert();
import array as arr
a = arr.array[‘i’,(4,5)]
a.append(6) # takes only 1 value as argument and adds it at the last
a = array('i', [4, 5,6])
a.extend([7,8,9]) # can take multiple values in 1 arguments in form of a list
a = array('i', [4, 5,6,7,8,9])
a.insert(2,10) # 1st argument specifies the index number and 2nd value
a = array('i', [4, 5,10,6,7,8,9])
-
What is the output of "Python is fun" [::-1] ?
Output : 'nuf si nohtyP'
-
What is the lambda function in python?
Lambda is an anonymous function that is defined without any name or the ‘def’ keyword. It is defined using the ‘lambda’ keyword. It can contain any number of arguments but only on expression. It is generally used when we require a nameless function for a short duration.
Syntax : lambda arguments : expression
Eg : a = lambda x : x**2
print(a(6))
Output : 36
-
If x = lambda a,b,c : (a+b)*c , what is the output of x(2,2,5) ?
a = 2 , b = 2 , c = 5 so (2 + 2) * 5 = 20.
-
What is the use of the split() function?
The split() function is used to separate the values of a string using the given delimiter and return it in a list. It takes 2 arguments separator and maxsplit. The separator is a delimiter using which string is split, its default delimiter is any white space. The maxsplit is the maximum number you want to split the string.
Eg: a = ‘Python is fun to learn.’
a.split(‘ ’,2)
Output : [‘Python’, ‘is’, ‘fun to learn.the’]
-
What is the difference between / and // in the division?
The / operator is used for the normal division while // is used for floor division i.e., // rounds the answer to the lower value.
Eg : 7 / 2 = 3.5
7 // 2 = 3
-
How can we convert a list of type strings to int?
We can use the map function.
a = [‘1’, ‘2’, ‘3’]
b = list(map(int, a))
Output = [1, 2, 3]
-
Can we multiply strings in Python?
Yes, multiplication of strings is possible in Python. When you multiply a string with k, you get a new string in which the original string is repeated k a number of times.
Eg: a = ‘hello’ * 3
Output: ‘hellohellohello’
-
What is the use of join()?
Join() takes all the items in an iterable as an argument and combines them into one string using the specified joining string.
Syntax: string.join(values)
Eg : a = [‘hello’, ‘world’]
“ % ”.join(a)
Output : hello % world
-
How can you swap 2 numbers without using a temporary variable?
Python allows 1 line code for swapping 2 numbers.
x, y = y, x
-
Write a program in Python to check if a string is palindrome.
a = input() if (a = a[::-1]): print(“palindrome”) else: print(“not palindrome”)
-
State any 5 functions of the math library.
Math library allows us to use various functions and constants to reduce the length of our code. Some of the functions are:
math. sqrt(): returns the square root of a number.
math.factorial(): returns the factorial of a number.
math.ceil(): rounds up a number to the nearest integer.
math.pow(): returns the value of x to the power of y.
math.pi: return the value of pi.
-
What are keywords? Name a few Python keywords.
In any language, keywords are reserved words that cannot be used for any user-defined variable or function. They are used for predefined or built-in identifiers, functions, or variables. Some of the python’s keywords are: if, else, break, and, is, class, finally, lambda, pass, etc. Python Online Course at FITA Academy clearly explains to you the concepts of the Pythons programming language and its applications in-depth under the training of Expert Python Developers.
-
What is the pass keyword used for in Python?
Pass is a null statement. It is used as a placeholder in place of a code. Execution of pass statement doesn’t give any output. It is used when the programmer doesn’t want to execute any code. Since empty code is not allowed in functions, loops, if statements, etc, the user can simply place a pass statement to avoid any error.
-
What are literals in python? Explain different literals in python.
Any data stored in a variable or a constant is known as literal. There are 4 kinds of literals in python:
String: These are sequences of characters enclosed within single, double or triple quotes. Character literals are single characters inside quotes.
Numeric: They are immutable and can be of any type integer, float, or complex.
Boolean: They can have only 2 possible values, either True or False. True is represented by 1 and False by 0.
Special: It is represented by ‘none’ for the values which have not been created.
-
What is concatenation? How can you concatenate 2 strings?
Concatenating means combining two objects to get a new object. Python allows a simple way to concatenate its objects. It can be done using the ‘+’ operator.
Eg: a = ‘hello’
b = ‘world’
print(a + “ ” + b)
Output : hello world
-
What are dictionaries in Python?
Dictionaries are an ordered collection of data objects. They are changeable and do not support duplicate values. The data is stored in the form of a key: value pair inside curly braces.
Eg: mydict = {
"name": "Alex",
"age": 24,
"city": “Mexico”
}
-
Explain the map function in Python.
Map() is a built-in function in Python that allows you to execute a specific function on each item of an iterable without using a loop. It takes 2 arguments, a function and an iterable. The function is executed on each item of the iterable.
syntax : map(function, iterable)
Eg: a = [2, 3, 4]
L = list(map( lambda x : x *2, a))
Output : [4, 6, 8]
-
What does time.time() return?
The time() function returns the number of seconds passed since January 1, 1970, 00:00:00 in a Unix system.
-
Write a short note in the pandas library.
Pandas is an open-source Python library. It is a fast, flexible, and powerful library used for data analysis and manipulation. It offers various data structures and data operations for manipulating numerical data and time series.
It can take input data from various sources such as CSV files, excel sheets, text files, etc. These are the commonly asked Python Interview Questions and Answers for Freshers and Experienced candidates.
-
Write a code snippet to clear a class in Python.
A class is created using the ‘class’ keyword.
class myClass: def __init__(self,city, state): self.city = city self.state = state p1 = myClass("Mumbai", “Maharashtra”) print(p1.city) print(p1.state) Output: Mumbai Maharashtra
-
How can you remove all whitespaces from a string?
Python offers a built-in function to delete all whitespaces from a string. It is strip().
Strip() delete all the preceding and trailing whitespaces in a string.
Eg: a = ‘ hello ’
a.strip()
Output: “hello”
-
How can you shuffle the items in a list?
The ‘random’ module offers a function called ‘shuffle()’ which rearranges the items in a list or array. Since shuffle() is a method of the random module, we first need to import random.
Eg : import random
a = [4,5,3,2,5]
random.shuffle(a)
print(a)
Output : [5, 4, 5, 3, 2]
-
What is break used for? Explain with an example.
The break statement is used to stop the execution of a block of code. It terminates the current code block and transfers the control to the next block outside.
Eg: a = [1,2,4,5,6,7,9] for i in a: if (i == 4): val = i Break print(i) print(“val found = ”, val) Output : 1 2 val found = 4
-
What is the use of help() and dir()?
The help() function is used to see the documentation of any function, keyword, class, or module. Its syntax is help(object), and the details of the object are displayed. If no argument is passed, the help’s interaction utility is opened on the console.
The dir() function is a built-in function in python which returns all the methods and attributes of any object. It even returns the built-in properties of an object.
-
What is the use of the len() function?
The len() function is used to determine the length of sequence data type objects like lists, tuples, arrays, strings, etc.
Eg: a = ‘hello’
print(len(a))
Output : 5
-
What are operators and operands?
An operator is a symbol that is used on some values to produce an output. Operators are used to performing operations on operands.
An operand is a value either numeric or some variable.
-
What are the different types of operators in Python with examples?
There are 3 kinds of operators in Python:
Unary: Operators which require a single operand. Eg: !a, -a
Binary: Operators which require two operands. Eg: a+b, a*b
Ternary: These require three operands. Eg: value = a if a < b else b
-
Why is Python known as an interpreted language?
Python is an interpreted language because it runs directly from the source code. The source code is converted to bytecode and then again translated to machine language depending upon the underlying operating system. Python code doesn’t require compilation before execution.
-
What is NumPy?
NumPy stands for Numerical Python. It is an open-source Python library that is used to work with arrays. It adds support for multi-dimensional arrays and matrices and also provides high-level mathematical functions for operating on these arrays.
-
Give any 3 reasons for choosing NumPy over lists?
NumPy arrays are preferred over lists for 3 reasons:
Uses less memory.
Up to 50 times faster than lists.
Convenient because of various supporting functions.
-
Is NumPy purely written in Python?
Although NumPy is a Python library, not all of its code is written in Python. Major parts of NumPy are written in C or C++ to meet the requirement of fast compilation.
-
How does the continued statement work?
The continued statement terminates the execution of the ongoing iteration of the statement being executed. It skips the rest of the code that follows the statement and starts with a new iteration in the loop.
-
Write a program to find the factorial of a number.
def fact(n): if n == 1: return n else: return n * fact(n-1)
-
What is exception handling?
An exception is a run time error that occurs during the execution of a program. To avoid the program from being interrupted due to the error that occurred, Python generates an exception during the execution that can be handled.
-
An identifier’s length can be up to how many characters?
a) 31 characters b) 63 characters
c) 79 characters d) None of these
An identifier can be of any length, therefore none of these.
-
What is the syntax of print statements in Python 2 and Python 3?
Python 2: print “Hello World”
Python 3: print(“Hello World”)
-
Write one line code to convert space separated user input to a list.
x = list( map( str, input().split()))
Input: 4 5 2 5 3 12 4
Output: [‘4’, ‘5’, ‘2’, ‘5’, ‘3’, ‘12’, ‘4’ ]
-
Explain different parts of exception handling.
An exception can be handled as follows:
Try: The error expected to occur will be tested in this block.
Except: The error can be handled here.
Else: This block will be executed if there is no exception.
Finally: This block will always get executed with or without exception.
-
What does type() return? What is the output of type(‘123’)?
The type() function returns the data type of the argument passed.
The output of the above code would be <class 'str'>.
-
When does the else part of try-except-else execute?
The else part is executed when there is no exception generated.
-
What is the ‘re’ module?
The ‘re’ module is a built-in Python package for RegEx or regular expression. RegEx is a sequence of characters that forms some pattern. It is used to check if a string contains the specified pattern.
-
Explain any 3 functions of the re module with examples.
The 3 functions are:
findall(): It returns a list containing all the matches. If no match is found, it returns an empty list.
Eg: import re
txt = "It is raining outside."
x = re.findall("in", txt)
print(x)
Output: [‘in’, ‘in’]
search(): It searches for a pattern in the string and returns the match object if the pattern matches. In the case of more than one match, it only returns the first occurrence. It returns None if no matches are found.
Eg: txt = “It is raining outside.”
x = re.search(‘n’, txt)
print(“the first n occurs at : ”, x.start())
Output : the first n occurs at : 9
split(): It returns a list where the string is split at every match.
Eg: txt = "It is raining outside."
x = re.split("\s", txt)
print(x)
Output: ['It', 'is', 'raining', 'outside.']
These are frequently asked Python Interview Questions and Answers for Freshers in an interview.
-
Consider a list x = [1, 2, 3, 4, 5, 6, 7, 8]. What will be the output after each of the following operations:
del x[4], remove(8), pop(2)?del x[4]: [1, 2, 3, 4, 6, 7, 8]
x.remove(8): [1, 2, 3, 4, 6, 7]
x.pop(2) : [1, 2, 4, 6, 7]
-
What is the os module? Can it be used to delete a file?
The os module is one of Python's standard utility modules. It provides functions to establish interaction between the user and the operating system. The methods of the os module are useful in manipulating files and directories.
The os module provides a remove() method which can be used to delete files or file paths.
Eg: import os
os.remove(‘abc.txt’)
It cannot be used to delete directories. If you want to delete an entire folder use rmdir().
Eg:os.rmdir(foldername)
-
What is GIL?
GIL is Python’s Global Interpreter Lock. It is a type of process lock which is used while dealing with processes. It allows only one thread at a time to control the execution i.e., only one thread will run at a particular time. Its impact cannot be seen in single-threaded programs but in the case of multi-threaded programs, it can be a performance bottleneck. GIL solves the problem of memory management by protecting the reference counter. It does this by adding locks to data structures that are shared about multiple threads. This is an important Python Interview Questions and Answers for Freshers and Experienced candidates.
-
Write a Python program to input 2 numbers can print their sum, difference, product, mod and division on different lines.
x,y = map( int, input().split()) print(“sum = ”, x+y, “\n”, “diff = ”, x-y, “\n”, “prod = ”, x*y, “\n”, “mod = ”, x%y, “\n”, “Div = ”, x/y) Input: x = 10 , y =2 Output: sum = 12 diff = 8 prod = 20 mod = 0 Div = 5
-
How is memory managed in Python?
Python manages its memory by using Python’s private heap space. The private heap space stores all the objects and data structures that are inaccessible to the programmer. Python’s memory manager allocates all Python objects to the private heap. The core API functions provide the programmer with some tools to code.
Python’s inbuilt garbage collector recycles all the unused memory and makes it available for the heap space.
-
What is PYTHONPATH?
Python’s environment variables greatly influence its behavior. One such environment variable is PYTHONPATH. It is used for setting the path for user-defined modules while importing them.
PYTHONPATH contains the presence of the imported modules in various directories, which is looked upon when importing a module. It is extremely useful in maintaining Python libraries that you don’t want to install in the global default location. Python Training in Bangalore at FITA Academy is a holistic training program that covers you extensively on the programming concepts and the important frameworks that are used in Python.
-
What is type conversion?
Converting one data type into another data type is known as type conversion.
int(): converts any data type into the integer type.
list(): converts any data type into a list.
hex(): converts integers into their corresponding hexadecimal numbers.
str(): converts any data type to string.
set(): returns the set of a sequence after converting it into a set.
-
Using for loops iterates over dictionaries.
d = {'name': ‘John’, 'age': 20, 'city': ‘Mexico’} for i, j in d.items(): print(i, '->', j) Output: name -> John age -> 20 city -> Mexico
-
What is the reference count?
The number of times an object is referenced by other objects in the system is known as a reference count. When an object’s references are removed, its reference count decreases. The system deallocates the object’s memory when its reference count becomes zero. This helps with memory management in Python.
-
What are data frames in Python?
Data frames are two-dimensional data structures provided by the pandas library in Python. They are two-dimensional, meaning they have a tabular structure containing rows and columns. They can store heterogeneous data across the two axes.
-
Is a b c = 10 10 10 a valid statement in Python?
The above statement is invalid as the Python variables cannot contain space between them.
a, b, c = 10, 10 , 10 is valid.
abc = 101010 is also valid.
-
What is Flask?
Flask is a micro web framework in Python. It is kind of a third-party library used for the development of web applications. Since Flask doesn’t require tools or libraries, it is classified as a micro-framework. It is a light framework as it does not depend on external libraries therefore fewer bugs.
-
Write a program to sort a nested list in descending order according to its 2nd element.
l = [[1,5], [5,2], [3,7], [8,1]]
l.sort(key = lambda x : x[1], reverse = True)
print(l)
Output : [[3, 7], [1, 5], [5, 2], [8, 1]]
-
What is the difference between x.reverse() and x[::-1]?
The former reverses a list without displaying any output while the latter displays the reserved list as output.
-
Create a data frame from a list.
A data frame can be created as follows:
import pandas as pd
l = [‘a’, ‘b’, ‘c’, ‘d’]
df = pd.DataFrame(l)
print(df)
Output : 0
0 a
1 b
2 c
3 d
4 e
-
State a few differences between Python and Java.
JAVA
PYTHON
It is a statically typed language.
It is a dynamically typed language.
It is compiled plus interpreted.
It is only interpreted.
Uses curly braces to define the beginning and end of a code block.
Uses indentation to specify code blocks.
Data types must be specified while declaring an object.
No use in declaring data types.
Eg: int a = 5;
Eg: a = 5
-
What are decorators in Python?
Decorators are extremely powerful tools in Python. They are useful in changing the behavior of an existing function or a class.
Decorators can add functionality to a function or class without changing their structure. They can even accept arguments for functions and modify those arguments before passing them onto the function.
In Python, they are called in bottom-up form and represented as @decorator_name.
-
What is set and frozenset?
Python provides 2 types of set data types: set and frozenset.
Type set can be modified after creation i.e., it is mutable and provides methods like add() and remove().
On the other hand, type frozenset is immutable and ordered.
-
Does the ‘=’ operator copies an object?
No, the ‘=’ operator is an assignment and does not copy an object. It only creates an attachment between the existing object and the new object.
If you want to create copies of an object, you have to use the copy module.
-
What is a shallow copy and deep copy? Explain with examples.
The copy module offers 2 kinds of copying methods:
Shallow copy: It contains the references of the objects to the original memory address. The changes made in the original copy are reflected in the new copy. It basically stores the copies of original objects and points references to objects.
Eg: from copy import copy
x = [1,2,[3,4],5]
x2 = copy(x)
x[3] = 7
x[2].append(6)
print(x2) # output => [1, 2, [3, 4, 6], 7]
print(x) # output => [1, 2, [3, 4, 6], 5]
Deep copy: It stores the copy of the original object value. The changes made in the copied object is not reflected in the original object
Eg: from copy import deepcopy
x = [1,2,[3,4],5]
x2 = deepcopy(x)
x[3] = 7
x[2].append(6)
print(x2) # output => [1, 2, [3, 4, 6], 7]
print(x) # output => [1, 2, [3, 4], 5]
These are commonly asked Python Interview Questions and Answers for Freshers and Experienced candidates.
-
What is serialization?
Serialization is the process of converting a data structure or data object into a stream of bytes. The object is stored or transmitted to a database, memory, or some file. The purpose of serialization is to save the state of an object so that it can be recreated whenever required.
-
Explain pickling and unpickling.
The serialization process in Python is known as pickling. It is the conversion of a Python object into a byte stream. The pickled objects can be compressed further and the process of pickling is also compact. Serialization is portable across versions. The process of pickling takes place through pickle.dump() function.
Unpickling is entirely the inverse of pickling. Here the byte stream is deserialized and converted back to the object. This process takes place through the pickle.load() function.
-
Write a one-liner code for joining the items of two lists.
a = [‘A’, ‘B’, ‘C’, ‘D’]
b = [‘a’ , ‘b’, ‘c’, ‘d’]
[‘’.join( [ i, j ] ) for i, j in zip (a , b)]
Output: [‘Aa’, ‘Bb’, ‘Cc’, ‘Dd’]
-
What does the zip() function do?
The zip() function takes iterables as arguments, joins them to form a tuple, and returns it. The zip() function maps the similar indexes of multiple objects so that they can be used as a single object.
Eg: name = [ "John", "Nik"]
age = [19, 25]
obj = zip(name, age)obj = set(obj)
print(obj)
Output : { ( 'John', 19 ), ( 'Nik' , 25 ) }
-
How can you unzip an object?
We can unzip an object by using the * operator.
Consider the above example:
name1, age1 = zip (*obj)
print(name1, age1)
Output: (‘John’, ‘Nik’) (19, 25)
-
Python provides how many types of file processing modes?
There are 4 file processing modes in Python: write-only, read-only, read-write, and append mode.
Read-only mode: ‘r’ is used to open a file in read-only mode. It is a default mode that opens a file for reading.
Write-only mode: ‘w’ opens the file in write-only mode. This mode opens a file for writing. Any data inside the file would be lost and a new file is created.
Read-Write mode: The file opens with ‘rw’.This is an updating mode as you can read as well as write in the file.
Append mode: ‘a’ opens the file in append mode. Any content written would be appended at the end of the file, if the file exists.
-
Explain different types of relational operators in Python.
Operators used for comparing the values are known as relational operators. They test the conditions and return a boolean value accordingly. The value can be either True or False.
Eg: x, y = 20, 12
print(x == y) # False
print(x < y) # False
print(y <= x) # True
print( x!=y ) # True
-
How can we get the first five and last five values of a data frame?
The head() function returns the value of the first 5 rows of a data frame written. The df.head() function returns top 5 entries by default. It can return the top n rows of a data frame by using df. head(n).
Similarly, the tail() function returns the last 5 entries of the data frame and df. tail(n) can fetch the last n values.
-
Write a program to open a file and display its contents?
with open(“file.txt”, ‘r’) as f:
fd = f.read()
print(fd)
-
Python supports the use of switch or case statements. True or False?
This statement is false.
-
What is the range function?
The range() function is used to generate a number list. It takes only integers as the argument, both positive and negative.
Syntax: range(start, stop, steps)
Here start gives the lower limit of the list, stop specifies the endpoint for the sequence and steps is the number of jumps to take between each number.
-
What does encapsulation mean?
Wrapping variables into a single entity is known as encapsulation. Like a class acts as a wrapper for the variables and functions. It is one of the fundamental concepts of object-oriented programming.
-
Give an example of abstraction.
Abstraction highlights only the usage of a function and hides its implementation for the user.
Eg: print( len ( [3,4,2,5] ) )
Output: 4
Here the user only gets the total length and not how the length was computed.
-
What will be the output of x * 3 if x = [1,2,3]?
The output will be [1, 2, 3, 1, 2, 3, 1, 2, 3]
-
Name the Python Libraries useful in a) machine learning b) scientific calculation c) neural networking.
Scikit-learn is used for machine learning, SciPy for scientific calculations, and Tensor Flow for neural networks.
These are persistent Python Interview Questions and Answers for Freshers in any interview.
-
Write a program to print all even numbers in a range.
n = int(input()) for i in range(n): if (i % 2 == 0): print(i)
-
What is a turtle?
Turtle is a built-in library in Python that is used for creating shapes and pictures when provided with virtual canvas. It is similar to a drawing board, which lets us command a turtle to get the desired picture.
We have tried to cover the most important and frequently asked questions in Python interviews in this article. We hope these questions and answers would be of great asset to you in cracking the interview you desire.
Apart from these preparation questions if you are willing to upskill your Python knowledge to ace that interview, check out Python Training in Chennai at FITA Academy. They provide extensive knowledge and training in python under the guidance of Python experts.