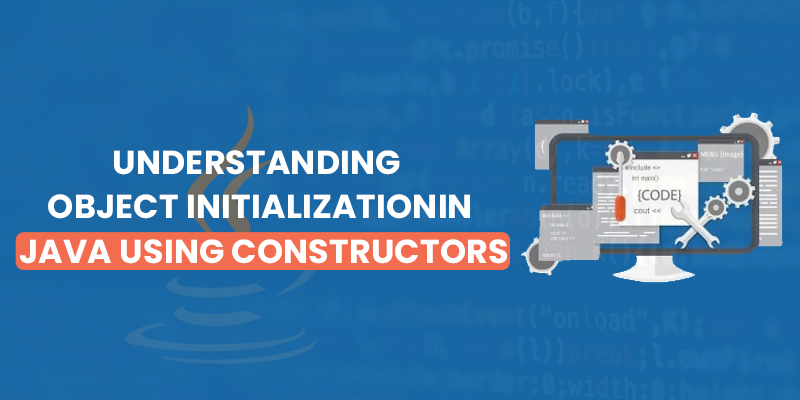
In the field of Java programming, object initialization is a fundamental concept that sets the groundwork for building robust and scalable applications. Constructors, as specialized methods, play a central role in initializing objects, ensuring their instantiation results in a valid and functional state. In this comprehensive blog, we will embark on a step-by-step journey to understand object initialization using constructor in java. Whether you're a developer with experience or just starting with Java, this blog will equip you with the essential knowledge and practical techniques to create well-structured, efficient, and maintainable code.
If you have a desire to learn Java and stay updated with its programming concepts in the latest technologies, Java Training in Chennai will guide you in gaining comprehensive knowledge of Java programming and explore its applications in latest technologies.
What is Object Initialization in Java?
Object initialization in Java refers to the process of creating an instance (object) of a class and setting up its initial state. When you define a class in Java, you are essentially creating a blueprint that describes the attributes (fields) and behaviors (methods) that objects of that class will have. However, a class by itself does not occupy memory or have a state; it is just a template.
Object initialization is the act of bringing that blueprint to life by creating an actual object in memory. When you create an object, memory is allocated to store the object's data, and the object's attributes are initialized to their default values (if not explicitly set) based on their data types. The constructor of the class is typically responsible for performing this initialization process. Here's how object initialization works in Java:
Example
Class Definition: First, you define a class with its attributes and methods.
public class Person {
private String name;
private int age;
// Constructor and other methods will be defined here...
}
Object Creation: To create an object of the class, you use the new keyword, followed by the class's constructor (if available) or the default constructor (if no explicit constructor is defined).
Person person1 = new Person();
Constructor Execution: When you call the constructor using the new keyword, memory is allocated for the object, and the constructor code is executed. The constructor initializes the object's attributes to their default values (if any) or sets them to the values provided in the constructor's parameters. For example, in the Person class, if a constructor with parameters is defined, you can initialize the object with specific values:
Person person2 = new Person("Alice", 25);
Object Initialization: After the constructor has executed, the object is fully initialized with its attributes set to the specified values. You can now use the object to access its attributes and call its methods.
System.out.println(person2.getName()); // Output: Alice
System.out.println(person2.getAge()); // Output: 25
Are you keenly interested in mastering Java and understanding object initialization? Fita Academy's Java Training in Coimbatore is an excellent choice where you can gain knowledge into Java programming and its important concepts like object initialization.
public class Person {
private String name;
private int age;
// Constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Getter for name
public String getName() {
return name;
}
// Setter for name
public void setName(String name) {
this.name = name;
}
// Getter for age
public int getAge() {
return age;
}
// Setter for age
public void setAge(int age) {
this.age = age;
}
// Example method
public void introduce() {
System.out.println("Hi, I'm " + name + " and I'm " + age + " years old.");
}
public static void main(String[] args) {
Person person1 = new Person("John", 25);
person1.introduce();
Person person2 = new Person("Alice", 30);
person2.introduce();
}
}
In the above example, the Person class has both a default constructor (with no parameters) and a custom constructor (with name and age parameters). These constructors initialize the name and age attributes of the Person object when it is created.
What is a Constructor?
A constructor resembles a method that is automatically called upon the creation of an object from a class. Unlike regular methods, a constructor shares the same name as the class and does not specify a return type. It serves the purpose of initializing the object's state and preparing it for use in the program. A Constructor is a basic component of a class which resembles a method but serves a distinct purpose. When an instance of the class is instantiated, the constructor is invoked, leading to the allocation of memory for the object in the system's memory space. Its primary role is to initialize the object, providing essential initial values or performing necessary setup operations.
Example
public class Person {
private String name;
private int age;
// Default constructor
public Person() {
name = "John Doe";
age = 30;
}
// Custom constructor with parameters
}
Syntax
public class ClassName {
// Instance variables and methods can be declared here
// Constructor declaration
public ClassName() {
// Constructor body (initialization code)
}
// Other methods can be defined here
}
Example of creating a simple class called Person with a constructor in java to initialize its attributes:
public class Person {
private String name;
private int age;
// Constructor with parameters
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Getter methods for the attributes
public String getName() {
return name;
}
public int getAge() {
return age;
}
public static void main(String[] args) {
// Creating objects using the constructor
Person person1 = new Person("Alice", 30);
Person person2 = new Person("Bob", 25);
// Accessing object attributes using getter methods
System.out.println(person1.getName() + " is " + person1.getAge() + " years old.");
System.out.println(person2.getName() + " is " + person2.getAge() + " years old.");
}
}
In this example, we have defined a Person class with two attributes: name and age. The constructor Person(String name, int age) takes name and age as parameters and initializes the object's attributes accordingly. The main method demonstrates how to create Person objects using the constructor and access their attributes using getter methods.
Role of Constructor in Object Initialization in Java
To know more information about why constructor is used in java, let’s say that Constructors are special methods specifically designed to facilitate the proper initialization of objects upon their creation, ensuring that they are in a valid and usable state right from the start. By incorporating constructors into our Java classes, we can enforce standardized and reliable object initialization procedures, enhancing the overall robustness and maintainability of our codebase.
Types of Constructors
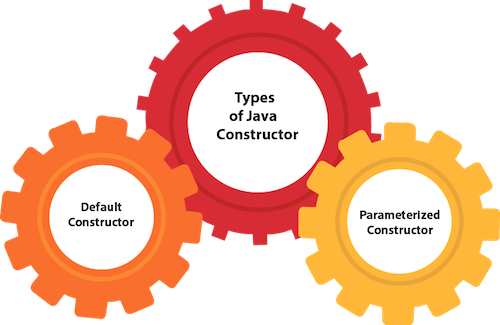
There are two types of constructor in java
- Default Constructor
- Parameterized Constructor
For those with a desire to learn Java and comprehend the role of constructors in initialization, Java Training in Bangalore helps you gain in-depth knowledge of Java programming and the crucial significance of constructors in the initialization process.
Default Constructor
In Java, constructors can be designed to accept parameters (arguments) or be defined without any parameters, referred to as a No-Argument or Default constructor. When you create a class in Java and do not explicitly define any constructors, Java automatically provides a default no-argument constructor for you. This default constructor initializes the object with default values (for primitive data types) or null (for reference data types).
Syntax
public class ClassName {
// Instance variables and other methods can be declared here
// No-argument constructor (Default constructor)
public ClassName() {
// Constructor body (initialization code, if needed)
}
// Other methods can be defined here
}
Example of No-Argument or Default Constructor
public class Car {
private String make;
private String model;
private int year;
// No-argument constructor
public Car() {
make = "Unknown";
model = "Unknown";
year = 0;
}
// Other methods can be defined here
public static void main(String[] args) {
// Creating an object using the no-argument constructor
Car myCar = new Car();
// Accessing object attributes
System.out.println("Make: " + myCar.make);
System.out.println("Model: " + myCar.model);
System.out.println("Year: " + myCar.year);
}
}
Parameterized Constructor
A parameterized constructor in java is a constructor that takes one or more parameters during its declaration. It allows you to initialize the object's attributes with specific values provided by the caller at the time of object creation.
Syntax
public class ClassName {
// Instance variables and other methods can be declared here
// Parameterized constructor
public ClassName(Type parameter1, Type parameter2, /* ... */) {
// Constructor body (initialization code using the provided parameters)
}
// Other methods can be defined here
}
Example of Parameterized Constructor
public class Student {
private String name;
private int age;
private String course;
// Parameterized constructor
public Student(String name, int age, String course) {
this.name = name;
this.age = age;
this.course = course;
}
// Other methods can be defined here
public static void main(String[] args) {
// Creating an object using the parameterized constructor
Student student1 = new Student("Alice", 20, "Computer Science");
Student student2 = new Student("Bob", 22, "Mathematics");
// Accessing object attributes initialized by the constructor
System.out.println("Student 1: " + student1.name + ", " + student1.age + ", " + student1.course);
System.out.println("Student 2: " + student2.name + ", " + student2.age + ", " + student2.course);
}
}
Constructors Overloading in Java
Constructor overloading in java refers to the practice of defining multiple constructors within a class, each having a different parameter list. Constructors can be overloaded in a similar way as function loading. This technique allows you to create objects with different sets of attributes or with varying initializations, providing flexibility in object creation based on different use cases. Overloaded constructors have the same name but different number o0of arguments.
Syntax
public class ClassName {
// Instance variables and other methods can be declared here
// Constructor with no parameters (default constructor)
public ClassName() {
// Constructor body (initialization code, if needed)
}
// Constructor with one set of parameters
public ClassName(Type parameter1, Type parameter2, /* ... */) {
// Constructor body (initialization code using the provided parameters)
}
// Constructor with a different set of parameters
public ClassName(Type parameterA, Type parameterB, /* ... */) {
// Constructor body (initialization code using the provided parameters)
}
// Other methods can be defined here
}
Example of Constructor Overloading
public class Rectangle {
private int length;
private int width;
// Constructor with no parameters (default constructor)
public Rectangle() {
length = 0;
width = 0;
}
// Constructor with two parameters
public Rectangle(int length, int width) {
this.length = length;
this.width = width;
}
// Constructor with one parameter (square)
public Rectangle(int side) {
this.length = side;
this.width = side;
}
// Other methods can be defined here
public static void main(String[] args) {
// Creating objects using different constructors
Rectangle rectangle1 = new Rectangle(); // Using the default constructor
Rectangle rectangle2 = new Rectangle(4, 6); // Using the constructor with two parameters
Rectangle square = new Rectangle(5); // Using the constructor with one parameter
// Accessing object attributes
System.out.println("Rectangle 1: Length = " + rectangle1.length + ", Width = " + rectangle1.width);
System.out.println("Rectangle 2: Length = " + rectangle2.length + ", Width = " + rectangle2.width);
System.out.println("Square: Length = " + square.length + ", Width = " + square.width);
}
}
If you are eager to learn Java and gain a deep understanding of programming concepts, especially in Object initialization,Java Training in Pondicherry at FITA Academy is the perfect choice for you. This course provides comprehensive training in Java programming, focusing specifically on the concepts of Object initialization.
Object initialization in Java involves creating an instance of a class and setting up its initial state by allocating memory, calling the constructor, and initializing the object's attributes. Object initialization is a fundamental step in Java programming and enables the use of objects to model real-world entities and perform tasks in a structured and organized manner. In this blog, we explored Constructors, its types, and why constructor is used in java. Additionally, we explored Constructor Overloading and Object Initialization in Java.