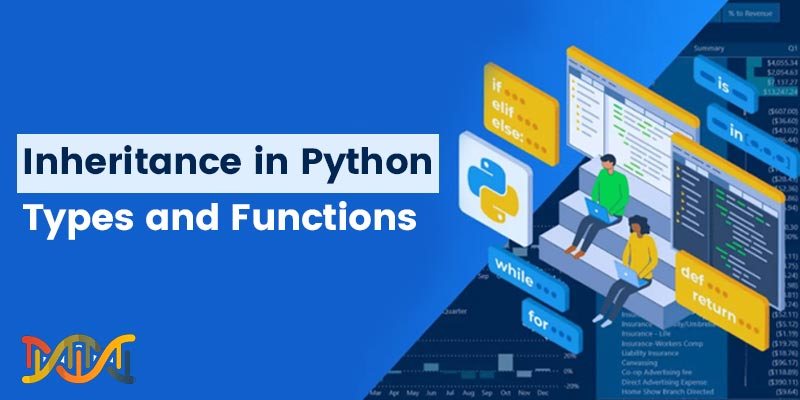
Python is a very popular and versatile programming language which is widely used by developers worldwide. It offers many functions and features that contribute to efficient code development and maintenance, including Hierarchical Organization, Polymorphism, Code Reuse, Code Maintenance, Overriding, and Extending Functionality. All these functions are achieved through the concept of Inheritance. It empowers the user to build scalable, modular, and efficient code structures. In this blog, we will explore the concept of inheritance in Python, its various types, and provide detailed examples. If you are looking to enhance your Python skills, you have come to the right place. FITA Academy offers top-notch Python Training in Chennai, where expert trainers with decades of experience will guide you from the basics to advanced levels using real-time examples and case studies.
Inheritance
Inheritance plays a vital role in promoting modularity, extensibility and code reusability in a hierarchical manner. Inheritance is a basic concept in Object-Oriented Programming that allows a class to inherit properties from another class. The main purpose of inheritance is to create a child class or derived class that inherits all the functions, data members, and properties from the parent class or base class. Instead of defining a new class from scratch, we can utilize an existing class to create a new class.
Child Class
Child class or a Derived class is a class that is derived from another class. In Python, creating a child class in inheritance involves defining a new class that extends or inherits the attributes and methods of the parent class.
Parent class
A Parent class or Base class is the class being inherited from. In Python, creating a parent class involves defining a class with its attributes and methods, which will serve as the blueprint for the child classes.
Syntax to define Parent class and Child class
# Parent class
class ParentClass:
# Parent class attributes and methods
# Child class inheriting from ParentClass
class ChildClass(ParentClass):
# Child class attributes and methods
Types of Inheritance
Inheritance types are categorized based on the number of child and parent classes involved. There are five types of inheritance.
- Single Inheritance
- Multiple Inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
Single Inheritance
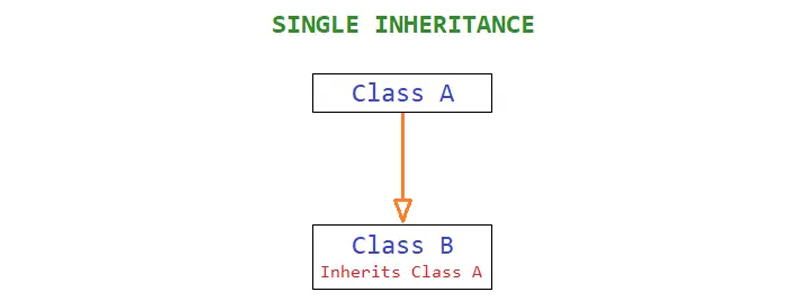
In single inheritance, the child class will be inherited from a single-parent class. The child class inherits all the properties, behaviors, attributes, and methods from a single parent class.
Syntax
class ChildClass(ParentClass):
# Class definition
Example of Single Inheritance
make_sound method of the Dog class my_dog.make_sound()
# Parent class
class Animal:
def __init__(self, name):
self.name = name
def make_sound(self):
pass
# Child class inheriting from Animal
class Dog(Animal):
def make_sound(self):
print(f"{self.name} says: Woof!")
# Create an instance of the Dog class
my_dog = Dog("Buddy")
# Call the make_sound method of the Dog class
my_dog.make_sound()
To know about what is single inheritance, let’s consider a parent class A and a child class B. Class B can inherit all the characteristics of Class A. It's like Class B is the offspring of Class A. By enrolling in the Python Course in Pondicherry, learners can gain knowledge and understanding of Python's fundamental concepts. The training program offers extensive guidance, enabling participants to grasp the core principles of Python effectively.
Multiple Inheritance
In Multiple inheritance, a child class acquires the properties and behaviors from multiple parent classes. Let's consider parent classes A and B, and a child class C. Class C can inherit abilities from both Classes A and B. It's like Class C has the combined qualities of Classes A and B, inheriting from multiple sources.
Syntax
class ChildClass(ParentClass1, ParentClass2, ...):
# Class definition
Example of Multiple Inheritance
# Parent class 1
class Animal:
def __init__(self, name):
self.name = name
def eat(self):
print(f"{self.name} is eating.")
# Parent class 2
class Bird:
def fly(self):
print("The bird is flying.")
# Child class inheriting from Animal and Bird
class Eagle(Animal, Bird):
def __init__(self, name):
super().__init__(name)
# Create an instance of the Eagle class
my_eagle = Eagle("Baldy")
# Call methods from both parent classes
my_eagle.eat()
my_eagle.fly()
Multilevel Inheritance
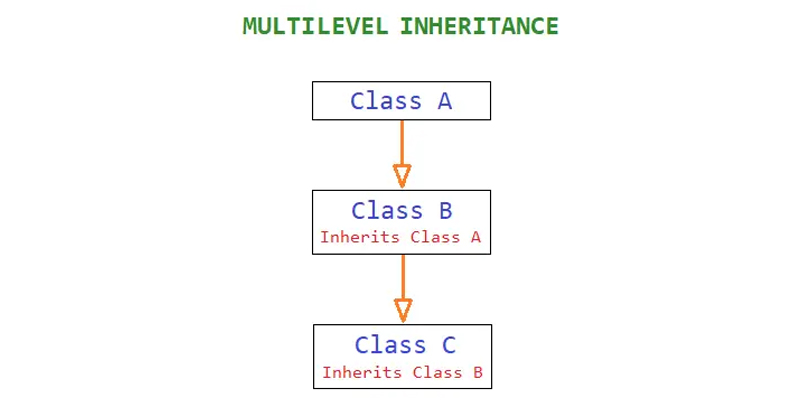
Multilevel inheritance in Python is a form of inheritance where a class is derived from a child class or a derived class. It is also known as a chain of classes. In Multilevel inheritance, a child class can acquire not only the attributes and methods of its immediate parent class but also the attributes and methods of the parent class's superclasses. If you seek Python courses to enhance your skills, look no further. By enrolling in a Python Course in Coimbatore, learners can gain a deeper understanding of new concepts in Python.
Syntax
class GrandparentClass:
# Grandparent class definition
class ParentClass(GrandparentClass):
# Parent class definition
class ChildClass(ParentClass):
# Child class definition
Example of Multilevel Inheritance
# Parent class
class Animal:
def __init__(self, name):
self.name = name
def eat(self):
print(f"{self.name} is eating.")
# Child class inheriting from Animal
class Dog(Animal):
def bark(self):
print(f"{self.name} is barking.")
# Grandchild class inheriting from Dog
class Puppy(Dog):
def wag_tail(self):
print(f"{self.name} is wagging its tail.")
# Create an instance of the Puppy class
my_puppy = Puppy("Max")
# Call methods from all ancestor classes
my_puppy.eat()
my_puppy.bark()
my_puppy.wag_tail()
Hierarchical Inheritance
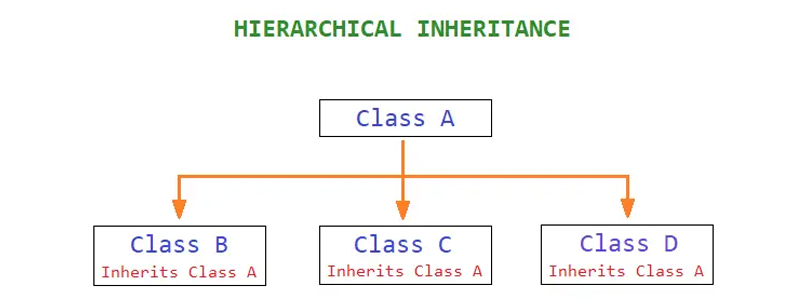
In Hierarchical inheritance multiple child classes are derived from a single parent class. It is one of the types of inheritance in which we have a parent class serving as the base, and several child classes branching out from it. Let’s think of a family tree where a single parent has multiple children. Each child acquires the characteristics and behaviors of the parent class by forming a hierarchy of classes.
Syntax
class ParentClass:
# Parent class definition
class ChildClass1(ParentClass):
# Child class 1 definition
class ChildClass2(ParentClass):
# Child class 2 definition
…
Example of Hierarchical Inheritance
# Parent class
class Animal:
def __init__(self, name):
self.name = name
def eat(self):
print(f"{self.name} is eating.")
# Child class 1 inheriting from Animal
class Dog(Animal):
def bark(self):
print(f"{self.name} is barking.")
# Child class 2 inheriting from Animal
class Cat(Animal):
def meow(self):
print(f"{self.name} is meowing.")
# Create instances of the Dog and Cat classes
my_dog = Dog("Buddy")
my_cat = Cat("Whiskers")
# Call methods from the respective classes
my_dog.eat()
my_dog.bark()
my_cat.eat()
my_cat.meow()
Hybrid Inheritance
Hybrid inheritance is a combination of different inheritance types in object-oriented programming. It allows a class to inherit attributes and methods from multiple parent classes. Think of hybrid inheritance as a blend of various inheritance types. It enables a class to inherit features from multiple parent classes, which results in a powerful inheritance structure. The syntax of hybrid inheritance depends on the specific combination of inheritance types used. Here is an example provided with a Hybrid inheritance program.
Example of Hybrid Inheritance
# Parent class
class Animal:
def __init__(self, name):
self.name = name
def eat(self):
print(f"{self.name} is eating.")
# Child class 1 inheriting from Animal
class Mammal(Animal):
def feed_milk(self):
print(f"{self.name} is feeding milk.")
# Child class 2 inheriting from Animal
class Bird(Animal):
def fly(self):
print(f"{self.name} is flying.")
# Grandchild class inheriting from both Mammal and Bird
class Bat(Mammal, Bird):
def __init__(self, name):
super().__init__(name)
# Create an instance of the Bat class
my_bat = Bat("Batty")
# Call methods from all ancestor classes
my_bat.eat()
my_bat.feed_milk()
my_bat.fly()
Python super() function
The super() function plays a crucial role in inheritance in Python. It allows you to refer to the parent class within a subclass, providing a way to customize and extend the functionality inherited from the parent. The subclass overrides a method defined in the superclass. By using super(), you can call and execute the overridden method from the superclass within the subclass. The expert mentors in Python Online Course will guide you by providing real-time examples and case studies, ensuring you acquire skills and knowledge in Python programming.
Syntax
class ChildClass(ParentClass):
def some_method(self):
super().method_name(arguments)
Example of super() function
class Animal:
def __init__(self, name):
self.name = name
def make_sound(self):
print("The animal makes a sound.")
class Cat(Animal):
def __init__(self, name, breed):
super().__init__(name)
self.breed = breed
def make_sound(self):
super().make_sound()
print("The cat meows.")
cat = Cat("Fluffy", "Persian")
cat.make_sound()
issubclass()
The issubclass() function is used for verifying the relationship between classes. It allows you to check if a certain class is a subclass of another class. If the class is a subclass of the specified class, the function will return True; otherwise, it will return False.
Syntax
issubclass(class, superclass)
Example of issubclass()
class Dog:
def bark(self):
print("The dog is barking.")
class Puppy(Dog):
def play(self):
print("The puppy is playing.")
# Check if Puppy is a subclass of Dog
print(issubclass(Puppy, Dog)) # Output: True
# Check if Dog is a subclass of Puppy
print(issubclass(Dog, Puppy)) # Output: False
Method Overriding
Overriding is a fundamental feature of inheritance in python that allows a child class to redefine a method inherited from its parent class. This gives the child class the ability to modify or extend the behavior of the parent class's method according to its specific requirements. The process of overriding involves matching the method name, parameters, and return type in the child class to those in the parent class.
Syntax
class ParentClass:
def method_name(self, arguments):
# Parent class method implementation
class ChildClass(ParentClass):
def method_name(self, arguments):
# Child class method implementation
Example program of Method overriding in Python
class Animal:
def sound(self):
print("The animal makes a sound.")
class Dog(Animal):
def sound(self):
print("The dog barks.")
class Cat(Animal):
def sound(self):
print("The cat meows.")
class Cow(Animal):
pass
animal = Animal()
dog = Dog()
cat = Cat()
cow = Cow()
animal.sound() # Output: The animal makes a sound.
dog.sound() # Output: The dog barks.
cat.sound() # Output: The cat meows.
cow.sound() # Output: The animal makes a sound.
Method Resolution Order in Python (MRO)
MRO is a concept in Python that determines the order in which methods or attributes are searched for within a class hierarchy. The MRO plays a crucial role in multiple inheritance scenarios, where a method with the same name exists in multiple parent classes.
- Method Resolution Order will search in the current parent class. If it is not available in the current parent class, then it searches in the parent class specified while inheriting.
- To get the MRO of a class, we can use either the mro attribute or mro method.
Syntax
ClassName.__mro__
Example of Method Resolution Order (MRO)
class A:
def foo(self):
print("A's foo")
class B(A):
def foo(self):
print("B's foo")
class C(A):
def foo(self):
print("C's foo")
class D(B, C):
pass
d = D()
d.foo()
print(D.mro())
Inheritance is an essential feature in Python that enables the establishment of hierarchical relationships between classes. It allows a child class to inherit attributes and methods from its parent class, promoting modularity and code reusability in OOPS. To enhance your understanding of Python programming, join the Python Training in Bangalore at FITA Academy. The training program offers a comprehensive learning experience, equipping you with the skills and knowledge required to excel in Python development.