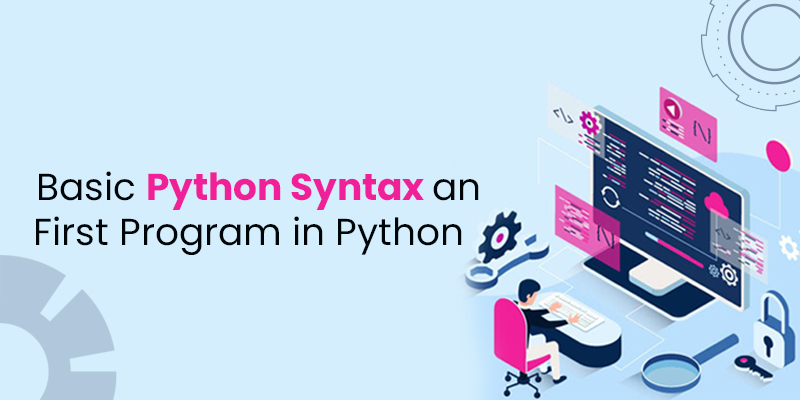
Python, recognised for its versatility and approachable learning curve, stands as a programming language renowned for its simplicity and code legibility. Its syntax employs indentation to delineate code blocks, offering code that is both concise and visually cohesive. This quality positions Python as a favoured choice for developers, whether they are newcomers or seasoned experts. One of the fundamentals we need to understand in order to code in any language is syntax. Indentation is a tool used in Python Syntax to denote a section of code. There are guidelines we must adhere to while using the indentation. We must adhere to the criteria for naming the variables as well as indentation. Learn Python Training in Bangalore from FITA Academy and start your journey to becoming a developer
Understanding Python Syntax
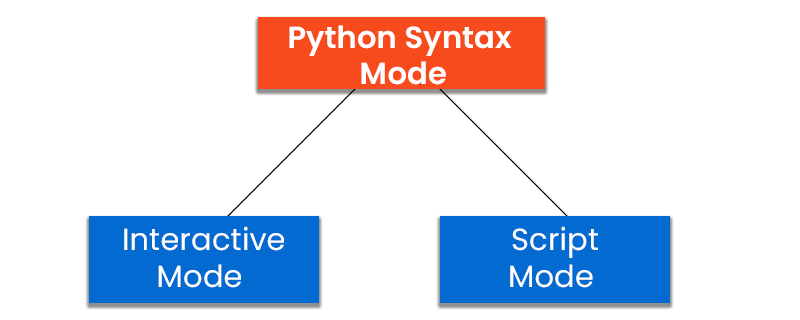
Before diving into Python syntax, it's essential to grasp how to create and execute a basic Python program, which can be accomplished in two ways:
- Interactive Mode: In this mode, you write and execute the program directly.
- Script Mode: In this mode, you create a .py file, save your code, and then run it.
To enter the interactive mode, open your terminal after installing Python and type:
$ python
You'll now be in the interactive mode, allowing you to execute Python statements directly. However, if you're using a script editor or integrated development environment (IDE), this step is unnecessary.
Now, let's delve into Python syntax, starting with a foundational element: the print statement.
Print Statement
Execute the following Python program in the terminal:
Python
>>> print("Basic Syntax of Python")
The output will be:
Basic Syntax of Python
Basic Syntax of Python
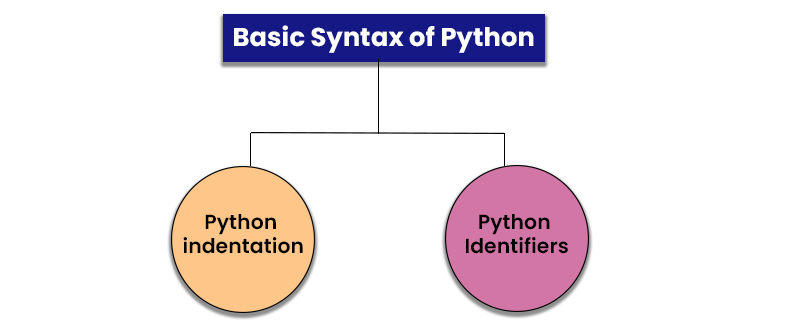
While we've covered the interactive mode, script mode offers another approach. To run code in script mode, create a .py file (e.g., syntax.py), write your code (including the print statement), and save it. Then, either use the 'run' button in your IDE or execute it in your terminal:
$ python syntax.py
Upon running the command, you'll observe the output: "Basic Syntax of Python" on the terminal. Congratulations, you've taken your first steps in understanding Python's fundamental syntax!
Python Indentation
When we write code in Python, such as functions or loops, we use a technique called "indentation" to specify blocks of code and determine their scope. This is achieved by adding a certain number of leading spaces or tabs before statements, and the general convention is to use one tab (or four spaces) for a single level of indentation.
To illustrate the concept of indentation levels, let's look at a simple example:
Python
def foo_bar():
print("Hello!")
if True:
print("Bye")
else:
print("See you soon")
print("Indentation in Python")
In this example, indentation levels play a crucial role in structuring the code. Let's break down the levels of indentation:
`def foo_bar():` marks the beginning of a function, so every line of code belonging to the function must be indented by at least one level. Notice that the `print` statements and the `if` statements are indented by at least one level. However, the last `print` statement is indented by 0 levels, indicating that it is not part of the function.
Inside the function block, which includes all statements indented below the function definition, we can see the following:
- The first `print` statement is indented by one level because it is directly under the function.
- The second `print` statement is further indented because it falls under the `if` statement.
When dealing with conditional statements like `if` and `else`, we follow specific rules for indentation:
- The `if` statement is indented by one level.
- Any code block under the `if` statement should be indented by one more level than the `if` statement itself.
- The same indentation rule applies to the `else` statement.
In Python, there are some important rules regarding code indentation:
- Indentation should not be split across multiple lines using the backslash character ('\\').
- Attempting to indent the first line of code will result in an `IndentationError`. The first line should not be indented.
- Maintaining consistent spacing throughout your code, either using tabs or spaces. Mixing tabs and spaces can lead to incorrect indentation.
- The preferred practice is to use one tab (or four spaces) for the first level of indentation and increment by four more spaces (or one more tab) for deeper levels of indentation.
Indenting code in Python offers several benefits, such as enhancing code readability and organisation. It provides a clear visual structure for understanding the code at a glance. Most modern integrated development environments (IDEs) automatically handle code indentation for you, simplifying the process.
Why Is Python Popular Among Developers? Understand the specialities and know about how easy it is to learn Python today!
However, one potential drawback of heavy indentation in extensive code is that fixing an indentation error in a single line can be challenging and time-consuming.
Let's examine some examples of indentation errors for better clarity:
Python
>>> y = 9
File "", line
y = 9
IndentationError: unexpected indent
>>>
In the above example, an `IndentationError` occurs because the first line is indented, which is not allowed in Python.
Python
if True:
print("Inside True")
print("Will throw an error due to extra whitespace")
else:
print("False")
This code will raise an `IndentationError` because the second `print` statement is indented with one level but has extra whitespace, which is not permitted in Python.
Python
if True:
print("Will throw an error due to 0 indentation level")
else:
print("Again, no indentation level, so an error will occur")
In the code above, the `print` statements inside the `if` and `else` blocks lack an additional level of indentation, leading to an `IndentationError: expected an indented Block`.
Python Identifiers
In Python, identifiers are names given to various code entities such as variables, functions, classes, or modules. These identifiers serve to differentiate one code entity from another. Here are some rules and guidelines for using identifiers in Python:
- An identifier can consist of a combination of lowercase (a-z) or uppercase (A-Z) alphabets, numbers (0-9), or underscores (_).
- An identifier cannot start with a digit. It must begin with an alphabet character (either lowercase or uppercase) or an underscore.
- Reserved words or keywords in Python cannot be used as identifier names. Keywords are special words with predefined meanings in the language (e.g., `if`, `else`, `for`, `while`, `import`, `class`, etc.).
- Symbols or special characters (such as !, @, #, $, %, etc.) cannot be used in an identifier.
- The length of an identifier can vary; there is no strict length restriction.
- Python is a case-sensitive language, so `var` and `VAR` are considered two different identifiers.
Here are some examples of correct and incorrect identifier naming:
- Correct Identifier Examples:
Python
var = 310
My_var = 20
My_var344 = 10
STRING = "PYTHON"
fLoat = 3.142
- Incorrect Identifier Examples:
python
97learning # Starts with a digit
hel!o # Contains a special character
Examples of functions with valid identifiers:
Python
def my_function():
print("Valid")
def My_function():
print("Valid")
def Myfunction3():
print("Valid")
def FUNCTION():
print("Valid")
To check if a particular name is a valid identifier in Python, you can use the built-in `is identifier ()` function. It can be used like this:
Python
print("var".isidentifier()) # Output: True
print("@var".isidentifier()) # Output: False
The first example returns `True` because "var" is a valid identifier, while the second example returns `False` because "@var" contains a special character and is not a valid identifier.
However, there is a caveat with the `is identifier ()` function: it will return `True` for any keyword in Python. Keywords cannot be used as identifiers, but this function does not distinguish between keywords and valid identifiers. Therefore, be cautious when using this function to check identifiers that might be keywords.
In Python, keywords are reserved for specific language constructs, and they serve predefined purposes in the language's syntax and semantics. Attempting to use keywords as identifiers in your code will result in syntax errors.
Variables in Python
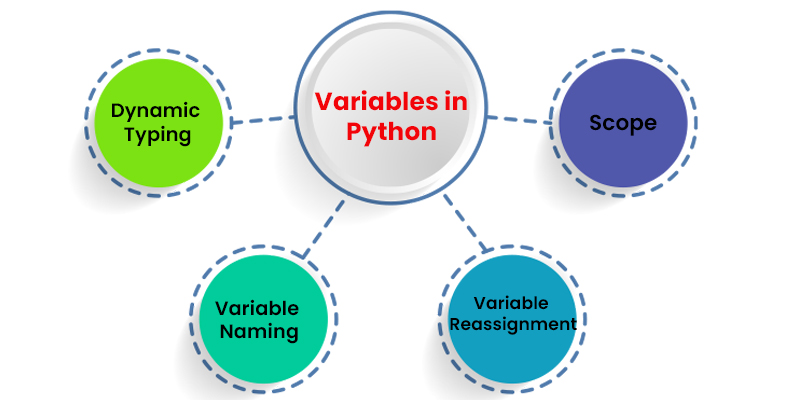
Variables are used in Python to hold data values or references to objects. Unlike some programming languages, where you need to explicitly declare the type of a variable, Python allows you to create variables dynamically by simply assigning a value to them. This feature is known as dynamic typing.
Here's how you can create and use variables in Python:
python
y = 9 # Assigning an integer value to the variable y
x = "Foo" # Assigning a string value to the variable x
print(x) # Printing the value of x
print(y) # Printing the value of y
The output of this code will be:
Foo
9
Join Python Training in Chennai and start your journey to becoming an AI/ML developer
Key points about variables in Python:
- Dynamic Typing: You don't need to declare the type of a variable explicitly. Python determines the type based on the value assigned to it. In the code above, `y` is an integer variable, and `x` is a string variable.
- Variable Naming: Variable names follow the rules of identifiers, as discussed in the previous section. They can contain letters (both uppercase and lowercase), numbers, and underscores. However, they cannot start with a digit, and reserved words or keywords cannot be used as variable names.
- Variable Reassignment: You can change the value of a variable at any time, and the variable's type can change as well. For example, you can change the variable `x` from a string to an integer or any other data type.
python
x = 42 # Changing the value of x to an integer
print(x) # Printing the updated value of x
- Scope: Variables have a scope that defines where in your code they can be accessed. Local variables are defined within a specific function or block of code, while global variables are accessible from anyplace in the script and defined outside of any function.
python
global_var = "I'm global" # A global variable
def my_function():
local_var = "I'm local" # A local variable
print(local_var) # Accessing the local variable
my_function()
print(global_var) # Accessing the global variable
Output:
I'm local
I'm global
Python's flexibility in variable handling allows you to write more concise and readable code, but it also requires careful consideration of variable names and their usage to avoid confusion and errors in your programs.
Join for FITA Academy Python Course in Pondicherry and be a pro at programming
Quotation in Python
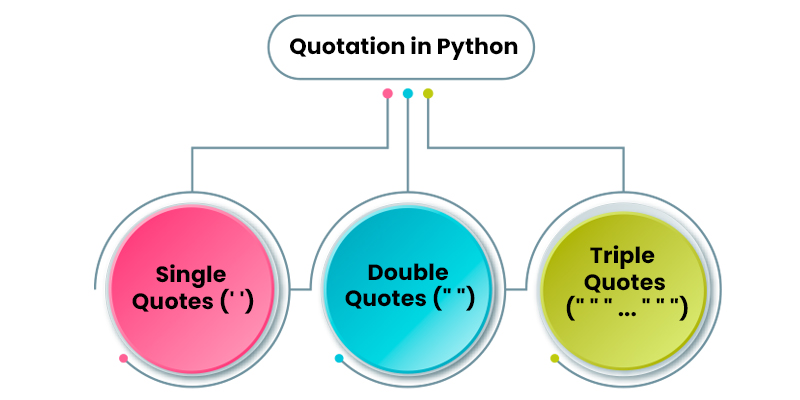
In Python, you can use three types of quotation marks to represent strings:
- Single Quotes (' '):
Single quotes are used to define strings in Python. You can enclose a sequence of characters within single quotes to create a string. For example:
```python
my_string = 'This is a string using single quotes.'
```
- Double Quotes (" "):
Double quotes can also be used to define strings. Like single quotes, you enclose a sequence of characters within double quotes to create a string. For example:
```python
my_string = "This is a string using double quotes."
```
Both single quotes and double quotes are interchangeable for defining strings in Python. Based on your preferences, you can select either style preference or to avoid conflicts with special characters within the string.
- Triple Quotes (" " " ... " " "):
Triple quotes (either single or double) are used to create multi-line strings in Python. They are also commonly used for docstrings, which are used to provide documentation for modules, functions, classes, or methods. Here's an example of a multi-line string:
```python
my_multiline_string = """
This is a multi-line string
in Python using triple quotes.
It can span across multiple lines.
"""
```
Triple quotes are useful when you need to include line breaks and special characters within your string without the need for escape characters.
Python provides flexibility in using either single or double quotes to define strings. Additionally, triple quotes are used for multi-line strings and docstrings. The choice of quotation marks depends on your specific requirements and coding style preferences.
Command-line arguments
Command-line arguments are a powerful way to make Python scripts more versatile and customisable. They allow you to pass data and options to your script when it's executed from the command line. This enables your script to work with different inputs, configurations, or settings without modifying the code.
To pass command-line arguments to a Python script, you can use the following format:
```bash
python script.py arg1 arg2 ... argN
```
Here, `script.py` is the name of your Python script, and `arg1`, `arg2`, ..., `argN` are the arguments you want to pass to the script.
To access and process these command-line arguments within your Python script, you can use the `sys` module, as you mentioned. Here's an example of how to do this:
```python
import sys
# The command-line arguments are available as a list in sys.argv
# The first element (sys.argv[0]) is the script name itself
# The following elements (sys.argv[1:], or sys.argv[1:]) are the arguments
arguments = sys.argv[1:]
# Now you can access and process the arguments
for arg in arguments:
print("Argument:", arg)
```
In this example, we import the `sys` module and use `sys.argv` to access the command-line arguments. The first element `sys.argv[0]` is the script's name, so we slice the list from `sys.argv[1:]` to access the actual arguments.
Here's how you would run this script and provide arguments:
```bash
python my_script.py arg1 arg2 arg3
```
The script would then process the provided arguments.
Command-line arguments are commonly used for tasks such as specifying file paths, configuration settings, input data, or any other information needed to customise the behaviour of your script. They make your scripts more flexible and adaptable to different scenarios and are a standard way to interact with command-line programs in Python. Enrol for the Python Online Course and learn in your free time achieving proficiency in the fundamentals of Python syntax and creating your first basic program in python marks a significant milestone in your programming education. We've explored essential concepts such as variables, data types, operators, and control structures, which serve as the foundational principles of Python programming. These basics are not only crucial for building your initial scripts but also for constructing more intricate, practical applications.
Regular and consistent practice is the cornerstone of becoming skilled in Python. By actively writing code, experimenting with various concepts, and taking on small-scale projects, you can cultivate and enhance your programming abilities. Python boasts a large and supportive community, providing an abundance of resources, tutorials, and online forums to assist you in your learning journey.
Armed with a strong understanding of basic code in Python and your basic program in python successfully executed, you're now ready to dive deeper into the various domains of Python programming. Whether your interests lie in web development, data analysis, machine learning, or any other field, Python's flexibility and readability make it an invaluable tool in your programming toolkit. Embrace your newfound knowledge, continue your learning journey by completing the basic program in python, and embark on your Python coding adventure!